So this is a quick introduction for machine learning with JavaScript.
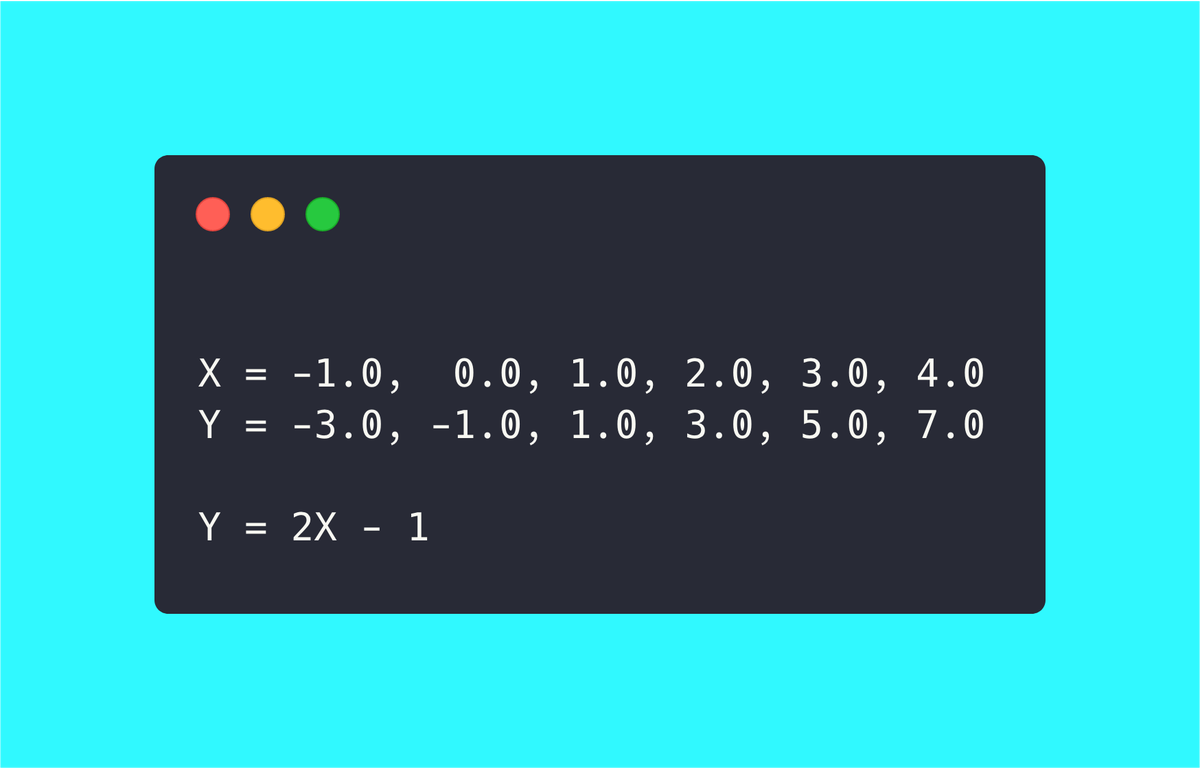
Above is a series of numbers. You can work out pretty easily that Y = 2X – 1. How you worked this out in your head is exactly how machine learning works.
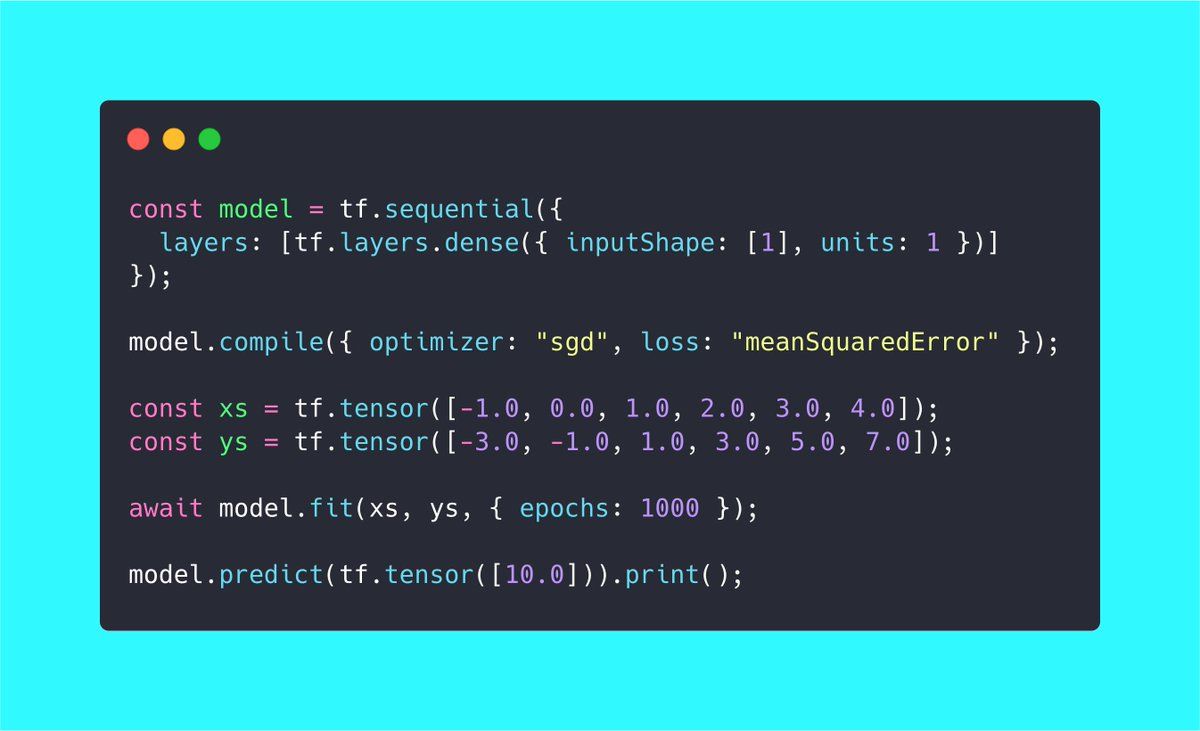
This is all the code you need to predict the pattern with machine learning and TensorFlow JS. Read on to learn what is happening here.
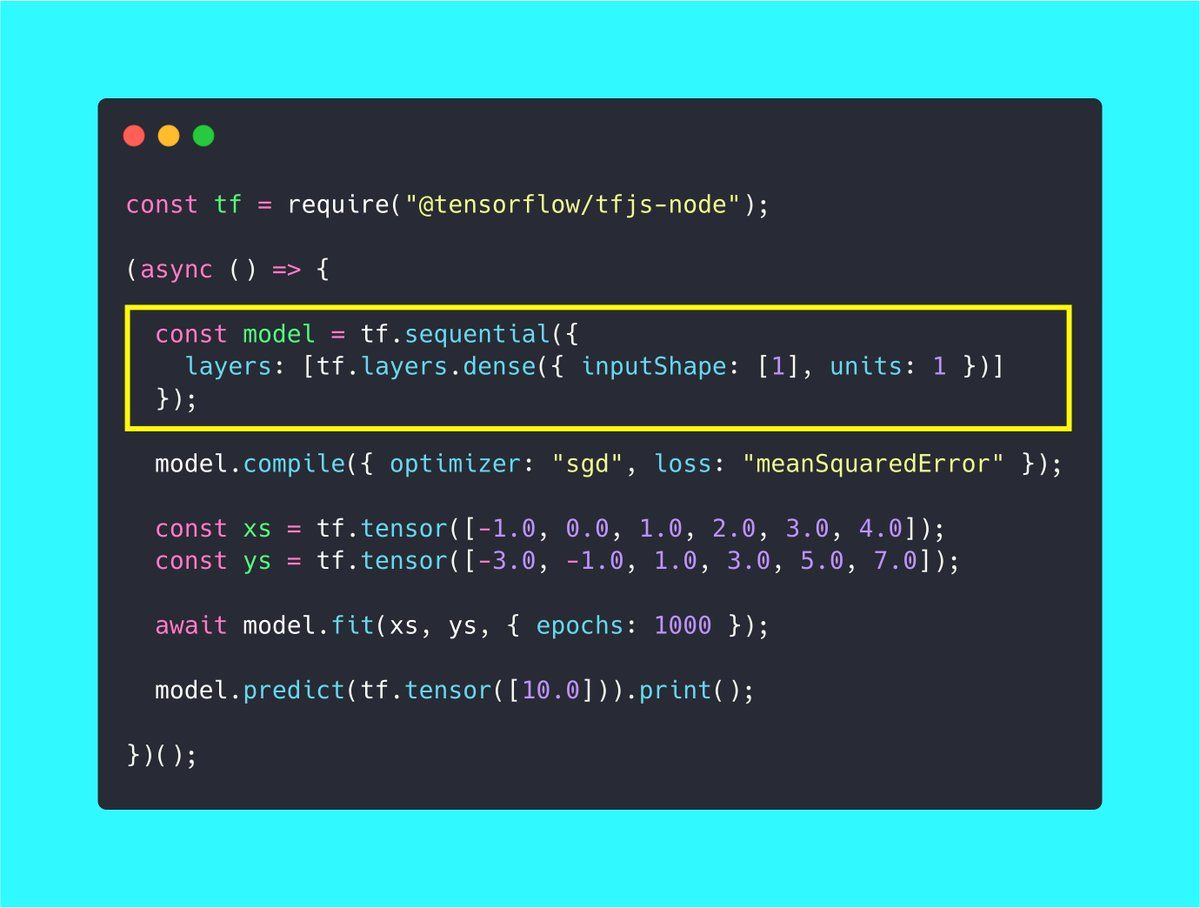
First up you will need to create a model. A model is a trained neural network. This is a basic model with a single layer. Our layer here is the method `tf.layers.dense`
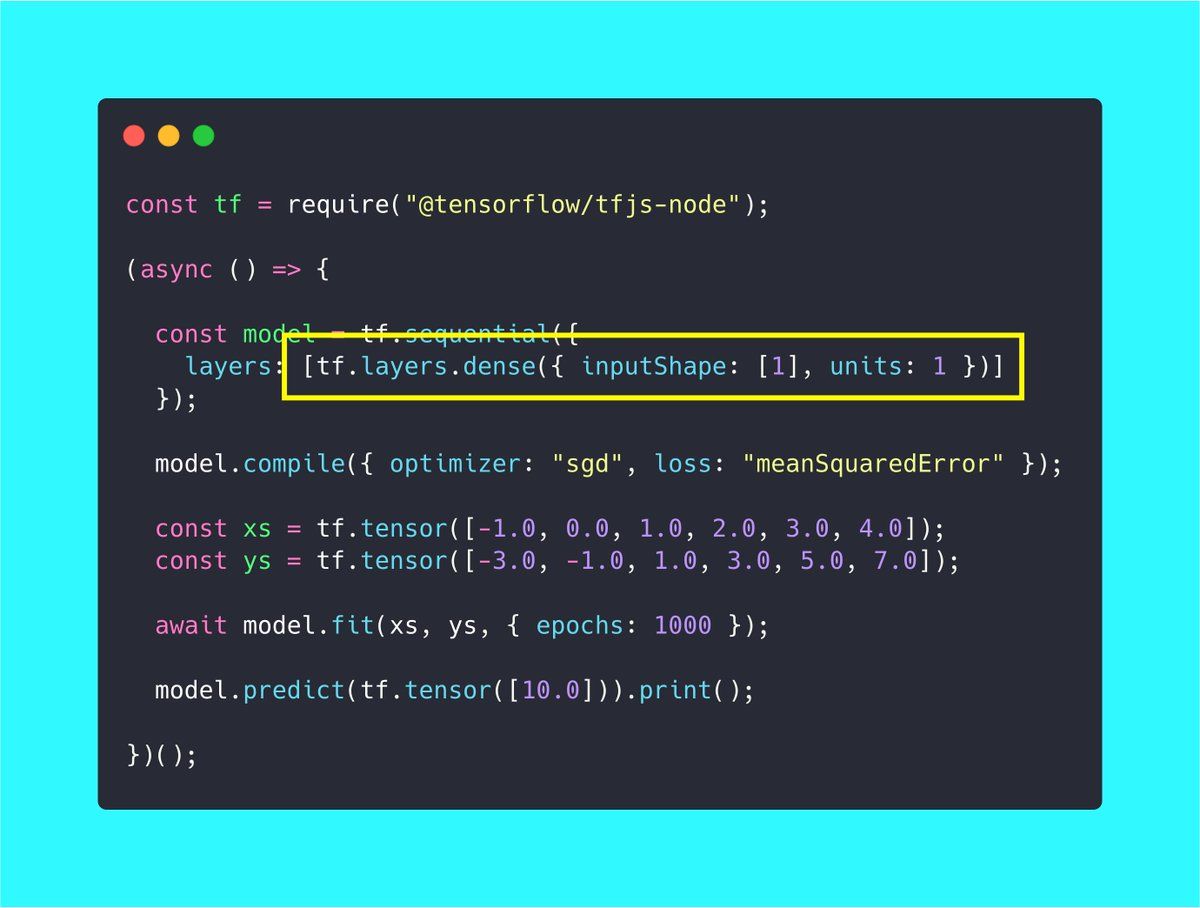
This layer has a single neuron which is represented by the property `units`. We are also feeding in a single value into the neural network represented by the property `inputShape`, which is our X value. Our neural network will try to predict the Y value.
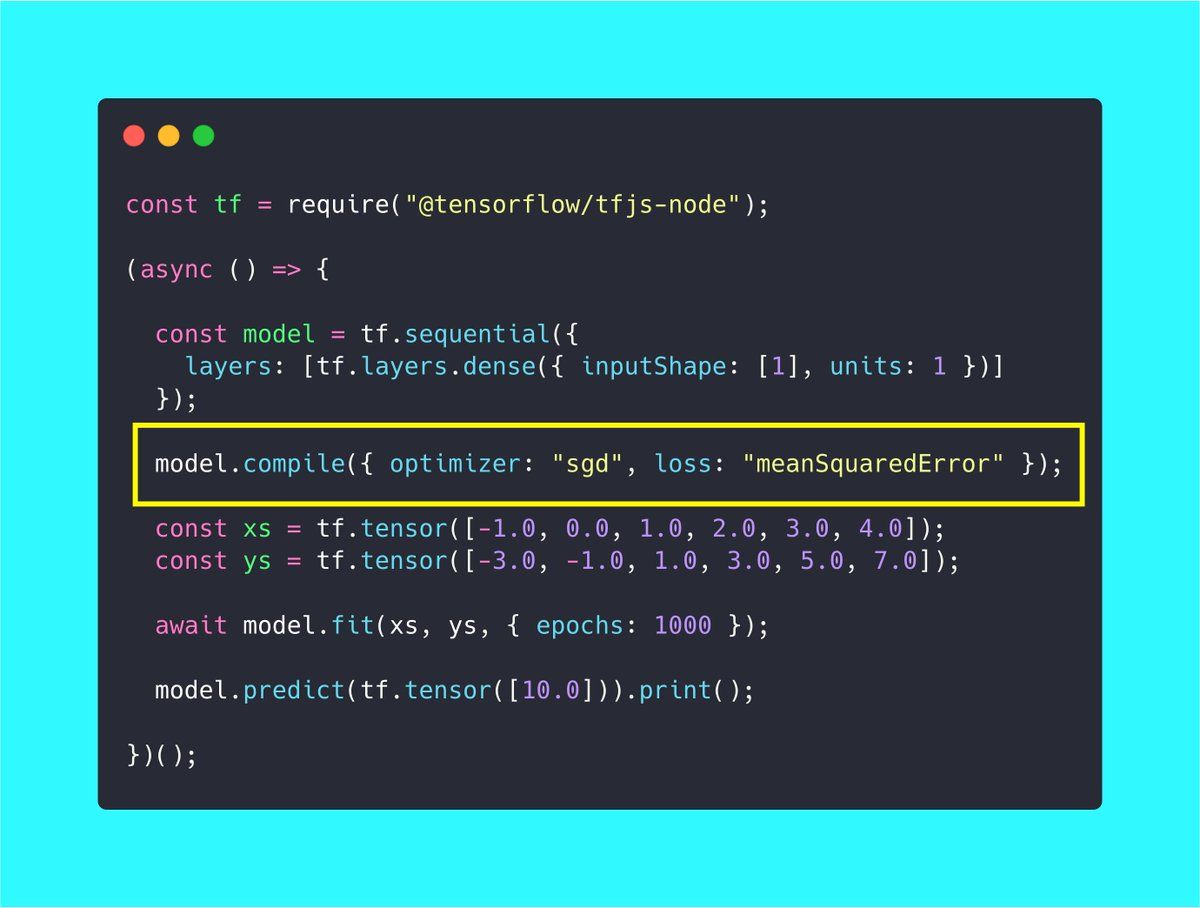
We need to compile our model. There are two main functions here. Loss and Optimizer. This is how our model learns. It will predict a number and the loss function will calculate how close it was. The Optimizer will predict the next guess and try to get closer to the right formula.
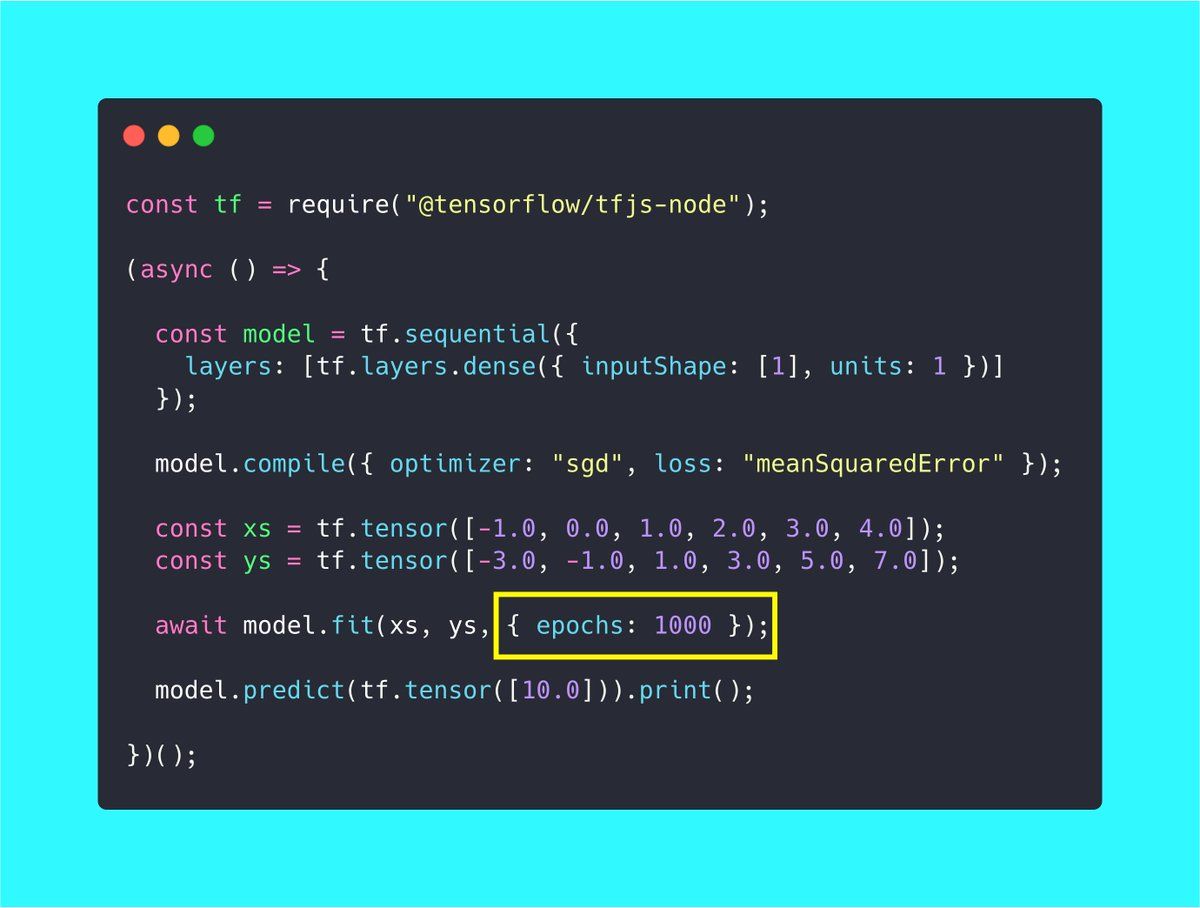
This will happen many times increasing our accuracy. In our example it will happen 1000 times, represented by the `epochs` property. Each time it will enhance its guess, getting us closer to the correct formula.
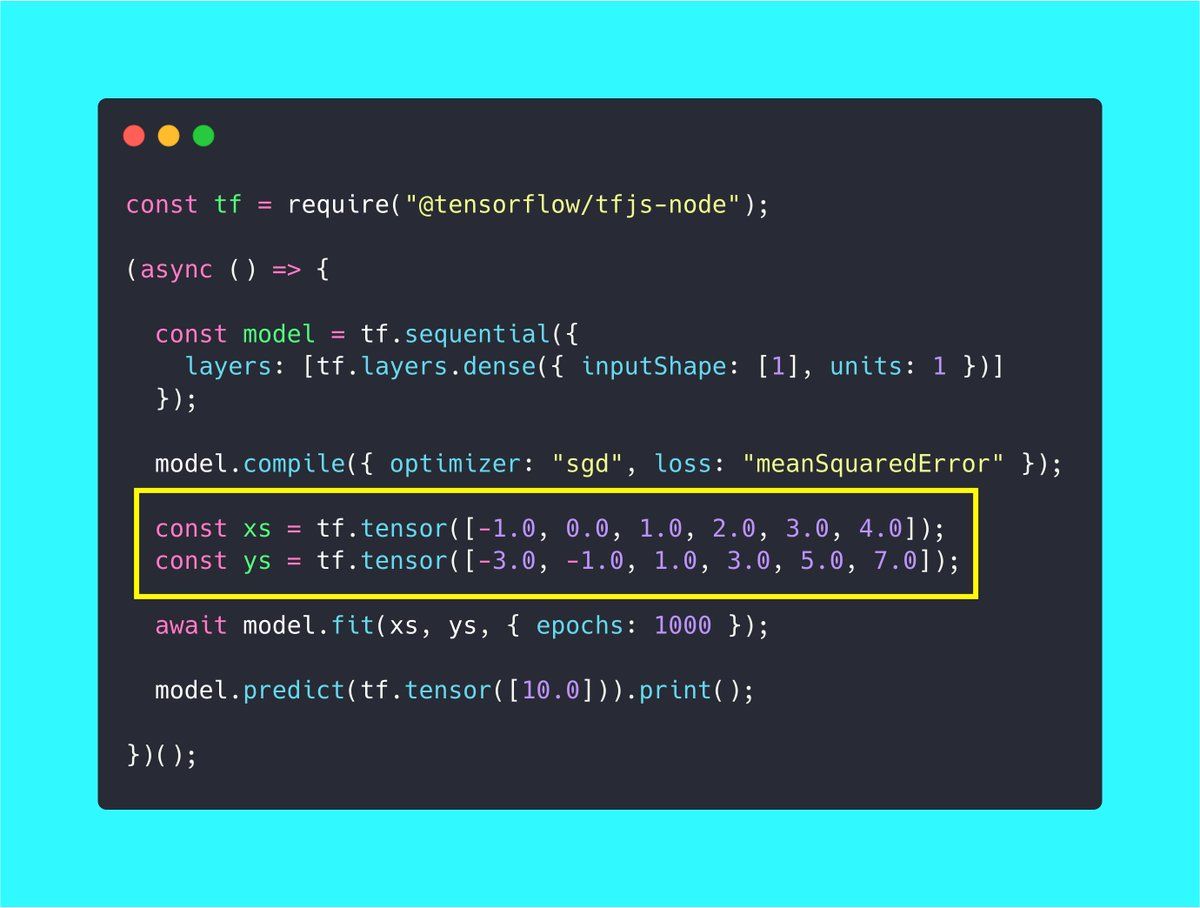
We have our two data sets represented as two arrays, `xs` and `xy`. We need to convert our data to tensors first using `tf.tensor`. This is just a holder for our data with type information.
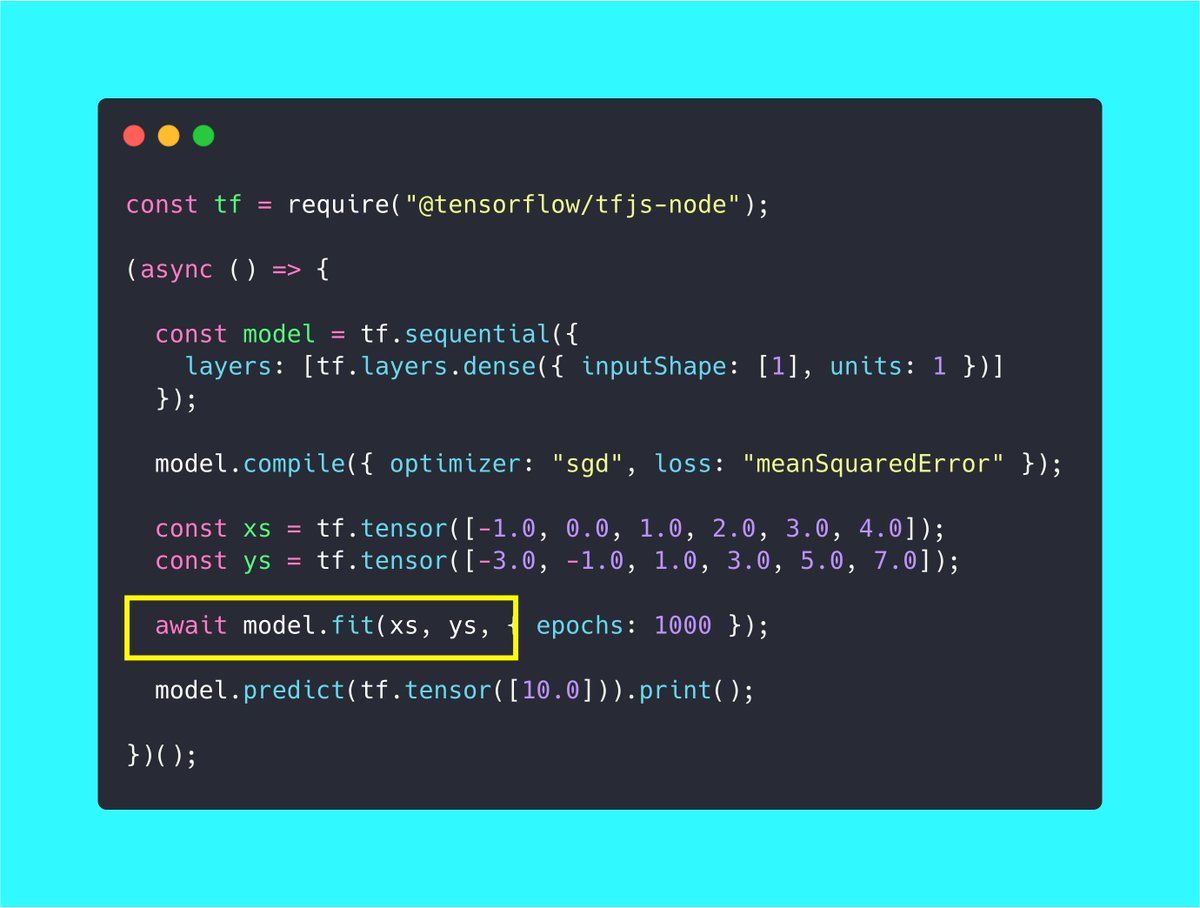
We match our two datasets to each other using the `model.fit` and passing in our two tensors (data). This method will take a while to complete, so we use `await` here.
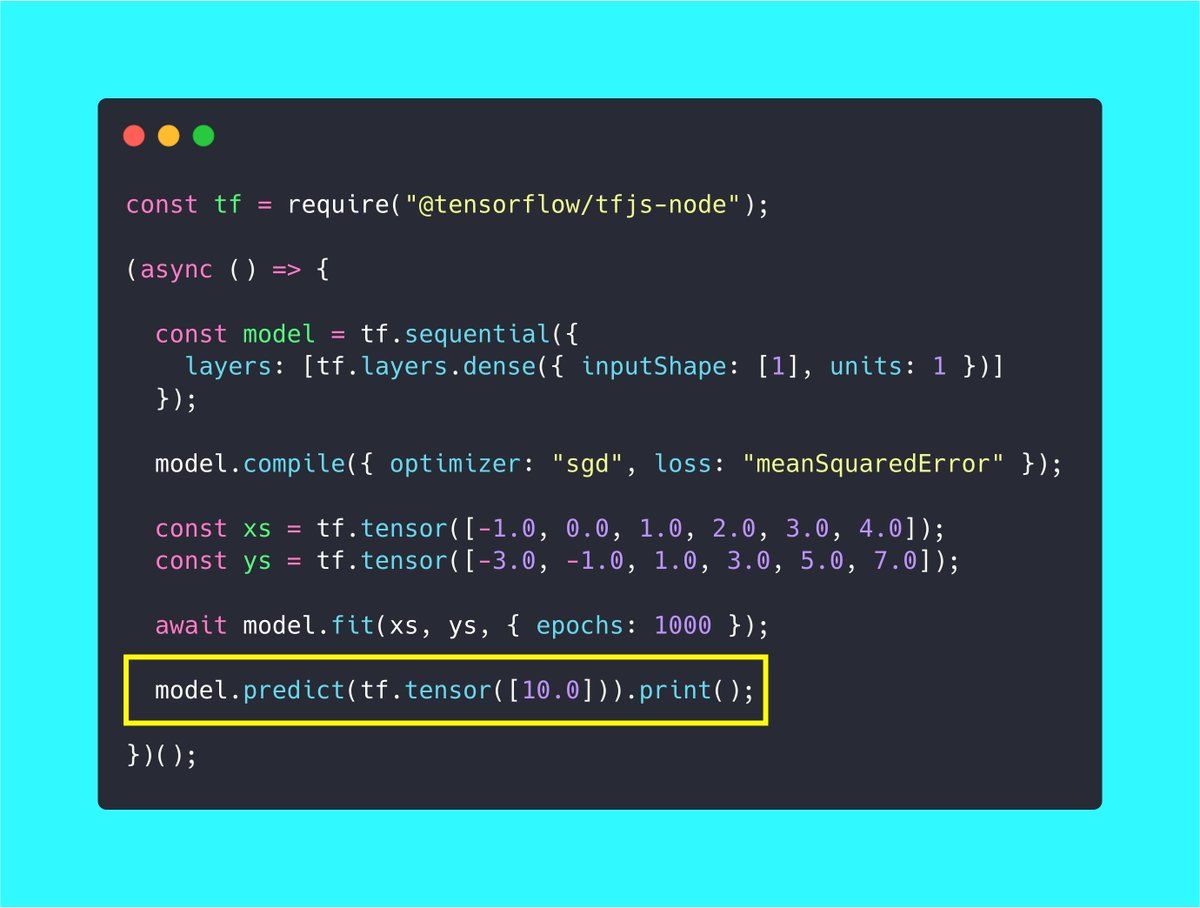
Once the model is trained. We can now predict with our model using `model.predict`.
So we are predicting the Y value for 10. We already know it will be 19 (Y = 2X – 1). When we run the following code our model gives us 18.999. Success!
You are probably wondering about the .999, this is because we are only giving it a small dataset and it is accounting for that in its prediction.
Hoped you like this article.
Below is the complete code.